Banner widget is a great thing to inform users about the environment in which the application works. For example, we want to show BETA
or TESTFLIGHT
ribbon for beta builds, just like the default DEBUG
banner warning is displayed when the app is built in debug mode.
Here is the method I’ve used to add BETA
badge.
/// Adds banner to the [child] widget.
Widget _wrapWithBanner(Widget child) {
return Banner(
child: child,
location: BannerLocation.topStart,
message: 'BETA',
color: Colors.green.withOpacity(0.6),
textStyle: TextStyle(
fontWeight: FontWeight.w700, fontSize: 12.0, letterSpacing: 1.0),
textDirection: TextDirection.ltr,
);
}
The usage is as simple as:
class MyApp extends StatelessWidget {
// This widget is the root of your application.
@override
Widget build(BuildContext context) {
return _wrapWithBanner(MaterialApp(
title: 'Flutter Demo',
theme: ThemeData(
primarySwatch: Colors.blue,
),
home: MyHomePage(title: 'Flutter Demo Home Page'),
));
}
...
}
Note I wrap th entire MaterialApp
, because I want the banner to be shown on every page of the app.
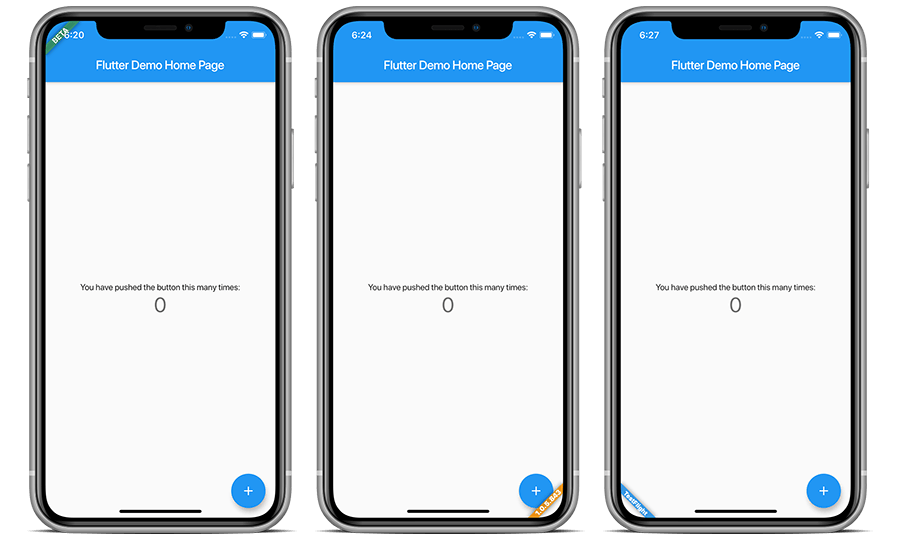
That’s it! Happy coding!